Introduction
JavaScript has come to be a power house in the web development world. And as it continues to evolve so are the techniques to milk it for the most. Here are the top 10 essential hacks every pro should know in 2024.
1. Destructuring Assignment
It is a process that makes it easier to get values from arrays or objects into variables. Now, all can be extracted at once instead of accessing each element or property one by one.
Example:
const user = { name: ‘John’, age: 30, job: ‘developer’ }; const { name, age, job } = user;
Pros | Cons |
Clean and readable code | Can become confusing with deeply nested objects. |
Reduces lines of code |
Pro tip: Combine with default values for added robustness.
2. Optional Chaining
Optional chaining allows you to safely access deeply nested properties without worrying about errors.
Example:
const user = { profile: { name: ‘John’ } };
console.log(user?.profile?.name); // John
console.log(user?.profile?.age); // undefined, no error
Pros | Cons |
Avoids runtime errors | Can hide issues in your data structure |
Makes code more resilient |
Pro tip: Use it with care, especially in critical code paths.
3. Nullish Coalescing Operator
This operator (??) helps to assign default values only when the left operand is null or undefined.
Example:
const name = user.name ?? ‘Guest’;
Pros | Cons |
More precise than `|| ` for handling defaults | Only works with `null` or `undefined ` |
Prevents unintended false values |
Pro tip: Great for default values in function parameters.
4. Template Literals
Template literals enhance string interpolation, making it easier to embed expressions.
Example:
const name = ‘John’;
const greeting = Hello, ${name}!
;
Pros | Cons |
Cleaner string formatting | Overuse can make code less readable |
Supports multi-line strings |
Pro tip: Use for dynamic HTML generation.
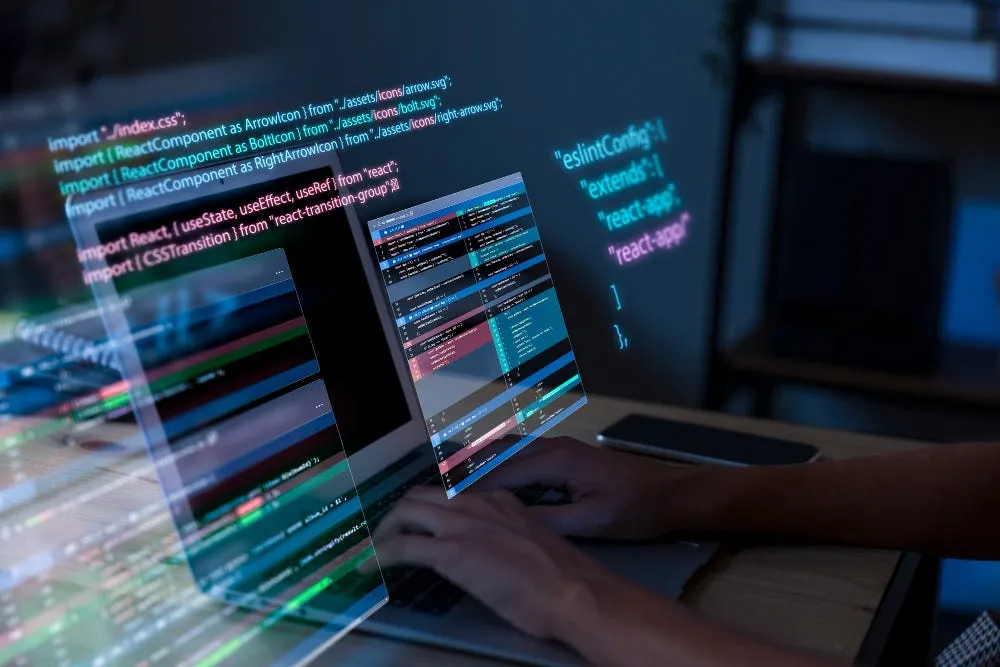
5. Async/Await Enhancements
Async/Await makes handling asynchronous code easier. In 2024, enhancements make it even more powerful.
Example:
async function fetchData() {
try {
const response = await fetch(‘https://api.example.com/data’);
const data = await response.json();
console.log(data);
} catch (error) {
console.error(‘Error fetching data’, error);
}
}
Pros | Cons |
Simplifies promise handling | Error handling can be tricky |
More readable than ".then() ” chains |
Pro tip: Use Promise.all
with async/await
for parallel execution.
6. Short-Circuit Evaluation
Short-circuiting lets you streamline conditions by leveraging logical operators.
const isLoggedIn = true;
const welcomeMessage = isLoggedIn && ‘Welcome back!’;
Pros | Cons |
Reduces code complexity | Can be confusing in more complex logic |
Improves performance |
7. Array Methods You Might Not Know
JavaScript arrays come with powerful methods that go beyond the basics.
Examples:
Array.prototype.flat()
: Flattens nested arrays.Array.prototype.reduce()
: Reduces an array to a single value.Array.prototype.find()
: Finds the first element that satisfies a condition.
Pros | Cons |
Reduces the need for loops | Some methods are less intuitive |
Makes code more declarative |
Pro tip: Master these methods to write more functional code.
8. Memoization Techniques
Memoization optimizes performance by storing the results of expensive function calls.
Examples:
const memoizedFunction = (() => {
const cache = {};
return (x) => {
if (cache[x]) return cache[x];
const result = x * 2; // Expensive calculation
cache[x] = result;
return result;
};
})();
Pros | Cons |
Improves performance | Increases memory usage |
Reduces redundant computations |
Pro tip: Use for functions with heavy computation.
9. Dynamic Imports
Dynamic imports let you load modules only when needed, improving performance.
Examples:
async function loadModule() {
const module = await import(‘./module.js’);
module.doSomething();
}
Pros | Cons |
Reduces initial load time | Can complicate debugging |
Enhances modularity |
Pro tip: Use for large libraries or rarely used features.
10. Proxy Objects
Proxy objects allow you to intercept and redefine fundamental operations.
Examples:
const handler = {
get: (obj, prop) => (prop in obj ? obj[prop] : ‘Not Found’),
};
const user = new Proxy({ name: ‘John’ }, handler);
console.log(user.name); // John
console.log(user.age); // Not Found
Pros | Cons |
Enables advanced operations | Can lead to unexpected behaviors |
Provides fine-grained control over object behavior |
Pro tip: Use proxies to add validation or logging.
Conclusion
Mastering these JavaScript hacks will elevate your code in 2024. They enhance readability, boost performance, and reduce bugs. Keep experimenting and refining your skills to stay ahead in the ever-evolving world of web development.
Related Post:
12 Best JavaScript Animation Libraries 2024
FAQs
Q: What is the most important JavaScript hack for 2024?
A: All the hacks listed are important, but optional chaining is a game-changer for handling nested properties safely.
Q: How can I implement these hacks in my current projects?
A: Start by refactoring small parts of your codebase to use these techniques. Over time, you can integrate them into your development process.
Q: Will these hacks work in all browsers?
A: Most of these hacks are well-supported in modern browsers, but always check compatibility for older versions.
1 thought on “10 JavaScript Hacks Every Pro Needs to Know in 2024”